
In this tutorial, I'm just referring from this link. See code below!
In this Example creating custom spinner with one image and two texts.
After spinner item selection showing selected item text on screen.
Steps :
1. Create Model (SpinnerModel.java) to store data for each spinner row.
2. Create a ArrayList to store Model (SpinnerModel.java) objects.
3. Store data in Models and Store Model objects in Arraylist.
4. Pass Model object Arraylist to custom adapter.
5. Custom Adapter use Arraylist data (Model Objects) and create rows for Spinner.
6. Create listener for Spinner and show spinner item selected values on activity.
2. Create a ArrayList to store Model (SpinnerModel.java) objects.
3. Store data in Models and Store Model objects in Arraylist.
4. Pass Model object Arraylist to custom adapter.
5. Custom Adapter use Arraylist data (Model Objects) and create rows for Spinner.
6. Create listener for Spinner and show spinner item selected values on activity.
Note :
Please read this tutorial first - Spinner Basics
Project Structure :
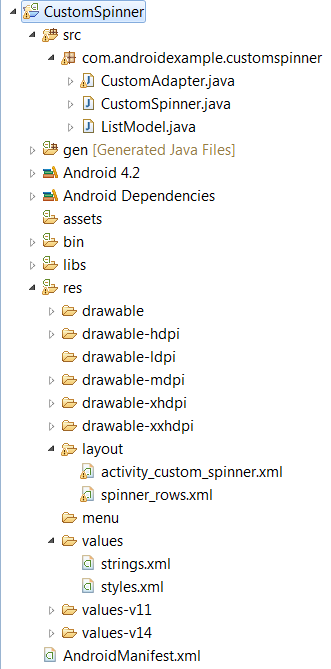
File : src/SpinnerModel.java
public class SpinnerModel { private String CompanyName=""; private String Image=""; private String Url=""; /*********** Set Methods ******************/ public void setCompanyName(String CompanyName) { this.CompanyName = CompanyName; } public void setImage(String Image) { this.Image = Image; } public void setUrl(String Url) { this.Url = Url; } /*********** Get Methods ****************/ public String getCompanyName() { return this.CompanyName; } public String getImage() { return this.Image; } public String getUrl() { return this.Url; } }
File : res/layout/activity_custom_spinner.xml
Define spinner and output text.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent"> <TextView android:paddingTop="20dip" android:layout_width="fill_parent" android:layout_height="wrap_content"/> <Spinner android:id="@+id/spinner" android:drawSelectorOnTop="true" android:prompt="@string/defaultText" android:layout_width="fill_parent" android:layout_height="wrap_content" /> <TextView android:paddingTop="20dip" android:paddingLeft="20dip" android:layout_width="fill_parent" android:layout_height="wrap_content" android:id="@+id/output" /> </LinearLayout>
File : src/CustomSpinner.java
Explanation i have commented in code.
import java.util.ArrayList; import android.app.Activity; import android.content.res.Resources; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.AdapterView.OnItemSelectedListener; import android.widget.Spinner; import android.widget.TextView; import android.widget.Toast; public class CustomSpinner extends Activity { /************** Intialize Variables *************/ public ArrayList<SpinnerModel> CustomListViewValuesArr = new ArrayList<SpinnerModel>(); TextView output = null; CustomAdapter adapter; CustomSpinner activity = null; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_custom_spinner); activity = this; Spinner SpinnerExample = (Spinner)findViewById(R.id.spinner); output = (TextView)findViewById(R.id.output); // Set data in arraylist setListData(); // Resources passed to adapter to get image Resources res = getResources(); // Create custom adapter object ( see below CustomAdapter.java ) adapter = new CustomAdapter(activity, R.layout.spinner_rows, CustomListViewValuesArr,res); // Set adapter to spinner SpinnerExample.setAdapter(adapter); // Listener called when spinner item selected SpinnerExample.setOnItemSelectedListener(new OnItemSelectedListener() { @Override public void onItemSelected(AdapterView<?> parentView, View v, int position, long id) { // your code here // Get selected row data to show on screen String Company = ((TextView) v.findViewById(R.id.company)).getText().toString(); String CompanyUrl = ((TextView) v.findViewById(R.id.sub)).getText().toString(); String OutputMsg = "Selected Company : \n\n"+Company+"\n"+CompanyUrl; output.setText(OutputMsg); Toast.makeText( getApplicationContext(),OutputMsg, Toast.LENGTH_LONG).show(); } @Override public void onNothingSelected(AdapterView<?> parentView) { // your code here } }); } /****** Function to set data in ArrayList *************/ public void setListData() { // Now i have taken static values by loop. // For further inhancement we can take data by webservice / json / xml; for (int i = 0; i < 11; i++) { final SpinnerModel sched = new SpinnerModel(); /******* Firstly take data in model object ******/ sched.setCompanyName("Company "+i); sched.setImage("image"+i); sched.setUrl("http:\\www."+i+".com"); /******** Take Model Object in ArrayList **********/ CustomListViewValuesArr.add(sched); } } }
File : src/CustomAdapter.java
Read comments in code for explanation
import java.util.ArrayList; import android.app.Activity; import android.content.Context; import android.content.res.Resources; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.ArrayAdapter; import android.widget.ImageView; import android.widget.TextView; /***** Adapter class extends with ArrayAdapter ******/ public class CustomAdapter extends ArrayAdapter<String>{ private Activity activity; private ArrayList data; public Resources res; SpinnerModel tempValues=null; LayoutInflater inflater; /************* CustomAdapter Constructor *****************/ public CustomAdapter( CustomSpinner activitySpinner, int textViewResourceId, ArrayList objects, Resources resLocal ) { super(activitySpinner, textViewResourceId, objects); /********** Take passed values **********/ activity = activitySpinner; data = objects; res = resLocal; /*********** Layout inflator to call external xml layout () **********************/ inflater = (LayoutInflater)activity.getSystemService(Context.LAYOUT_INFLATER_SERVICE); } @Override public View getDropDownView(int position, View convertView,ViewGroup parent) { return getCustomView(position, convertView, parent); } @Override public View getView(int position, View convertView, ViewGroup parent) { return getCustomView(position, convertView, parent); } // This funtion called for each row ( Called data.size() times ) public View getCustomView(int position, View convertView, ViewGroup parent) { /********** Inflate spinner_rows.xml file for each row ( Defined below ) ************/ View row = inflater.inflate(R.layout.spinner_rows, parent, false); /***** Get each Model object from Arraylist ********/ tempValues = null; tempValues = (SpinnerModel) data.get(position); TextView label = (TextView)row.findViewById(R.id.company); TextView sub = (TextView)row.findViewById(R.id.sub); ImageView companyLogo = (ImageView)row.findViewById(R.id.image); if(position==0){ // Default selected Spinner item label.setText("Please select company"); sub.setText(""); } else { // Set values for spinner each row label.setText(tempValues.getCompanyName()); sub.setText(tempValues.getUrl()); companyLogo.setImageResource(res.getIdentifier ("com.androidexample.customspinner:drawable/" + tempValues.getImage(),null,null)); } return row; } }
File : res/layout/spinner_rows.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="vertical" android:padding="3dip" > <ImageView android:id="@+id/image" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:layout_toRightOf="@+id/image" android:padding="3dip" android:layout_marginTop="2dip" android:textColor="@drawable/red" android:textStyle="bold" android:id="@+id/company" android:layout_marginLeft="5dip" android:layout_width="wrap_content" android:layout_height="wrap_content"/> <TextView android:layout_toRightOf="@+id/image" android:padding="2dip" android:textColor="@drawable/darkgrey" android:layout_marginLeft="5dip" android:id="@+id/sub" android:layout_below="@+id/company" android:layout_width="wrap_content" android:layout_height="wrap_content"/> </RelativeLayout>
File : res/values/strings.xml
<?xml version="1.0" encoding="utf-8"?> <resources> <string name="hello">Custom Spinner</string> <string name="defaultText"> Select your Company </string> <string name="app_name">CustomSpinner Demo</string> <drawable name="Blue">#b0e0e6</drawable> <drawable name="white">#ffffff</drawable> <drawable name="black">#000000</drawable> <drawable name="green">#347C2C</drawable> <drawable name="pink">#FF00FF</drawable> <drawable name="violet">#a020f0</drawable> <drawable name="grey">#cccccc</drawable> <drawable name="red">#C11B17</drawable> <drawable name="yellow">#FFFF8C</drawable> <drawable name="PowderBlue">#b0e0e6</drawable> <drawable name="brown">#2F1700</drawable> <string name="select_Category">Select Company</string> <drawable name="darkgrey">#606060</drawable> </resources>